Calculator Project
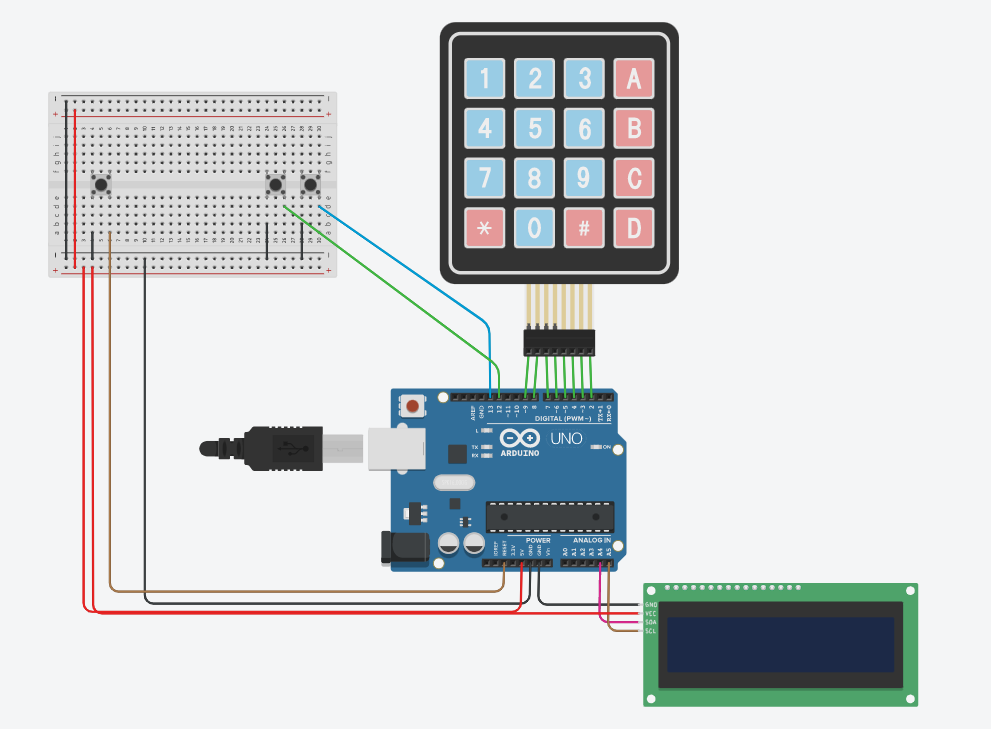
Four-Function Calculator
EE4953 Project | Fall 2022
GitHub Repository
Team Members
- Dominic Benintendi – Parser/Lexer development, system architecture
- Devin Macy – LCD/keypad logic, input handling, shift/button mappings
- Colin Russell – Documentation, user guide, interface design
- AL-Husain Bani Oraba – Diagrams, system requirements, documentation support
Overview
Features
- Arithmetic: Add, subtract, multiply, divide
- Extra operations: Parentheses, exponents, floating point
- Syntax error handling with helpful messages
- Expression editing with arrow keys, insert/delete
- History navigation for past expressions
- Dual clear functionality:
Clear
andClear All
Hardware
- Microcontroller: Arduino Nano
- Display: 1602 LCD via I2C
- Input: 4x4 membrane keypad + 2 shift pushbuttons
- Power: 5V via USB Mini-B
Keypad Mapping
- Mode Switching: Due to the limited number of keys on the keypad, mode switching was used
- Default Mode:
A–D
→+ - * /
, adjacent key →=
, lower left →Clear
- Left Shift Mode (L): Arrow keys,
del
,ins
- Right Shift Mode (R):
()
,.
,^
,Clear All
Software
- Input parsing: Lexer/tokenizer and recursive descent parser
- Computation: Infix notation with proper order of operations
- Display logic: Shows expressions and results with context-aware alignment
- Error handling: Returns messages like
ERR:SYNTAX
,ERR:DIV BY 0
, etc.
System Design
Modules:
CalcLexer
– Tokenizes user inputCalcParser
– Recursively evaluates expressionsLcdController
– Manages LCD contentKeypadController
– Maps key inputs and shift states
Architecture: Clean object-oriented design with clearly divided subsystems for input, display, and computation.
Build Diagrams
- TinkerCAD schematic of the system
- Breadboard and wiring layouts
- LCD and keypad pin connections
- Flowcharts for data processing and system interaction
Operating Notes
- Operates in 40%±30% humidity and –40°F to 185°F
- Not resistant to drops or moisture—no protective chassis
- Powered exclusively via USB
Expression Evaluation: Lexer & Parser
To support full arithmetic expressions (including parentheses, exponents, and floating-point numbers), we developed a custom recursive descent parser backed by a lexer/tokenizer. This allowed us to move beyond simple two-number operations and handle full algebraic expressions with correct operator precedence and error detection.
🔍 Lexer (Tokenizer)
+
, -
, *
, /
, ^
), parentheses, and the end of the expression.
Token types included:
NUM
: Any numeric value (integer or float)ADD
,SUB
,MUL
,DIV
,POW
: Arithmetic operatorsL_PAR
,R_PAR
: Left and right parenthesesEND
: End of expression
Example:
Input: "5 / (6 + 2)"
Tokens: NUM DIV L_PAR NUM ADD NUM R_PAR
🧠 Parser (Recursive Descent)
Operand:
→ **NUM**
EXP:
→ Operand
| **LEFT_PAR** ADD_SUB_EXP **RIGHT_PAR**
| **SUB** ADD_SUB_EXP
POW_EXP:
→ EXP **POW** EXP
| EXP
MUL_DIV_EXP:
→ POW_EXP **MUL** POW_EXP
| POW_EXP **DIV** POW_EXP
| POW_EXP
ADD_SUB_EXP:
→ MUL_DIV_EXP **ADD** MUL_DIV_EXP
| MUL_DIV_EXP **SUB** MUL_DIV_EXP
| MUL_DIV_EXP
This approach supports:
- Proper precedence:
*
and/
before+
and-
, and^
before both - Associativity: Left-to-right for most ops, right-to-left for
^
- Parentheses for grouping
- Negative numbers and unary minus (e.g.,
-5
,2 + -6
) - Floating point precision with 2-decimal accuracy on LCD
🛠 Error Handling
The lexer/parser combination detects and reports:
ERR:SYNTAX
– Invalid token arrangementERR:DIV BY 0
– Division by zeroERR:DECM SYNTAX
– Multiple decimal points in a numberERR:NO R_PAR
– Missing closing parenthesis
🧪 Sample Evaluations
Input: 5 + 3 * 5 → Output: 20 (Multiplication before addition)
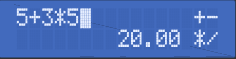
Input: (5 + 3) * 5 → Output: 40 (Parentheses override precedence)
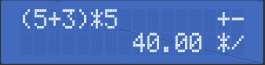
Input: 5 * ((4 - 5)/0.5) → Output: -10 (Nested expressions + float division)
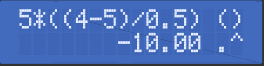